首页 > 编程笔记
C# IndexOf()方法:查找字符串第一次出现的位置
在 C# 中,IndexOf() 方法返回的是搜索的字符或字符串首次出现的索引位置,它有多种重载形式,其中常用的几种语法格式如下:
如果找到字符或字符串,则结果为 value 的从 0 开始的索引位置;如果未找到字符或字符串,则结果为 -1。
例如,查找字符 e 在字符串 str 中第一次出现的索引位置,代码如下:
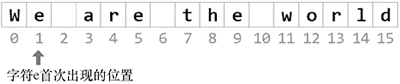
图 1 字符串str的下标排列
在日常开发工作中,经常会遇到判断一个字符串中是否包含某个字符或者某个子字符串的情况,这时就可以使用 IndexOf() 方法判断获取到的索引位置是否大于等于 0。如果是,则表示包含;否则,表示不包含。
再举个例子,查找字符串“We are the world”中“r”第一、二、三次出现的索引位置,代码如下。
public int IndexOf(char value) public int IndexOf(string value) public int IndexOf(char value,int startIndex) public int IndexOf(string value,int startIndex) public int IndexOf(char value,int startIndex,int count) public int IndexOf(string value,int startIndex,int count)
- value:要搜索的字符或字符串。
- startIndex:搜索起始位置。
- count:要检查的字符位置数。
如果找到字符或字符串,则结果为 value 的从 0 开始的索引位置;如果未找到字符或字符串,则结果为 -1。
例如,查找字符 e 在字符串 str 中第一次出现的索引位置,代码如下:
string str = "We are the world"; int size = str.IndexOf('e'); //size 的值为 1理解字符串的索引位置之前要对字符串的下标有所了解。在计算机中,string 对象是用数组表示的,字符串的下标是
[0, 数组长度-1]
。上面代码中的字符串 str 的下标排列如下图所示。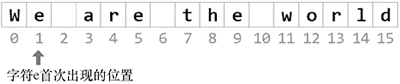
图 1 字符串str的下标排列
在日常开发工作中,经常会遇到判断一个字符串中是否包含某个字符或者某个子字符串的情况,这时就可以使用 IndexOf() 方法判断获取到的索引位置是否大于等于 0。如果是,则表示包含;否则,表示不包含。
再举个例子,查找字符串“We are the world”中“r”第一、二、三次出现的索引位置,代码如下。
static void Main(string[] args) { string str = "We are the world"; //创建字符串 int firstIndex = str.IndexOf("r"); //获取字符串中r第一次出现的索引位置 //获取字符串中r第二次出现的索引位置,从第一次出现的索引位置之后开始查找 int secondIndex = str.IndexOf("r", firstIndex + 1); //获取字符串中r第三次出现的索引位置,从第二次出现的索引位置之后开始查找 int thirdIndex = str.IndexOf("r", secondIndex + 1); //输出3次获取的索引位置 Console.WriteLine("r第一次出现的索引位置是" + firstIndex); Console.WriteLine("r第二次出现的索引位置是" + secondIndex); Console.WriteLine("r第三次出现的索引位置是" + thirdIndex); Console.ReadLine(); }程序运行结果为:
r第一次出现的索引位置是 4
r第二次出现的索引位置是 13
r第三次出现的索引位置是 -1